Search Filter in Angular 9
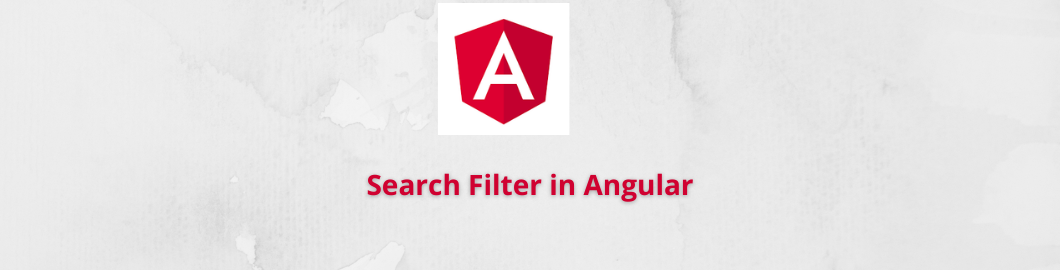
Search Filter in Angular 9
In this blog I will explain how can you create search filter in Angular 9.To do this you have to follow following steps :
Step1: create filter using following command
ng g pipe cateName --skipTests --module=app.module
If more than one module in your project you have to specify module name using [--module=app.module] --module .
Step2:Write following code in cateName pipe
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'cateName'
})
export class CateNamePipe implements PipeTransform {
transform(value: any, args: string): [] {
if(args)
{
args=args.toLowerCase();
return value.filter(function(el:any){
console.log(el)
return el.Name.toLowerCase().indexOf(args)>-1
})
}
return value;
}
}
Step3: write following html
<input type="text" [(ngModel)]="queryString" id="search" placeholder="category name">
<table border="2">
<thead>
<tr>
<td>Name</td>
<td>Description</td>
<td>
<a routerLink="add" >Add</a>
</td>
</tr>
<tr *ngFor="let c of categoryList | cateName:queryString">
<td>
{{c.Name | uppercase}}
</td>
<td>
{{c.Description}}
</td>
<td>
<a routerLink="edit/{{c.Id}}" >
<i class="fa fa-pencil"></i>
Edit</a>||
<a (click)="delete(c.Id)">Delete</a>
</td>
</tr>
</thead>
</table>
Step4: Write following code in Component.ts file
import { Component, OnInit } from '@angular/core';
import { Category } from 'src/app/Models/category.model';
import { CategorySService } from 'src/app/Services/category-s.service';
@Component({
selector: 'app-categories',
templateUrl: './categories.component.html',
styleUrls: ['./categories.component.css']
})
export class CategoriesComponent implements OnInit {
categoryList;
queryString
constructor(public service:CategorySService) { }
ngOnInit(): void {
this.loadCategory();
}
loadCategory(){
this.service.getAll().subscribe(s=>{
this.categoryList=s;
})
}
}
CategorySService is a service class that is responsible to manipulate record of Sal SERVER database ,using WebAPI core.
Step5: Run application using following command
Ng serve –o
It will display following output
Back to Blogs